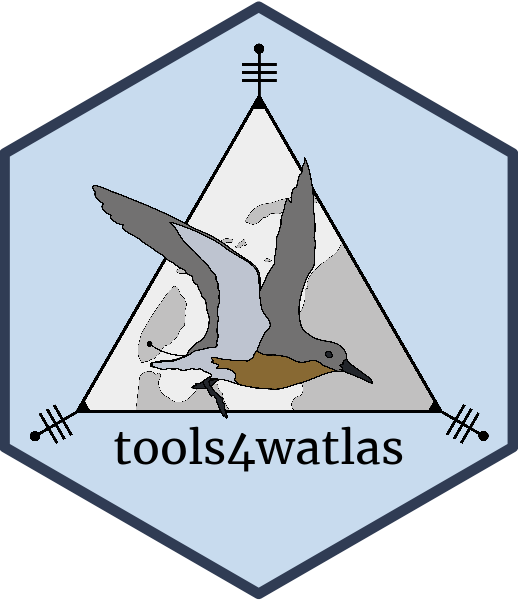
Plot data in loop
Johannes Krietsch
Source:vignettes/visualization_tutorials/plot_data_in_loop.Rmd
plot_data_in_loop.Rmd
This article shows how to make and save plots in a loop and parallel
loop using the package foreach
and doFuture
. This is useful for example when
checking the data by tag ID using atl_check_tag()
.
Plot and save data in loop
Example of a simple loop by tag ID and using
atl_check_tag()
.
# load example data
data <- data_example
# file path
path <- "./outputs/maps_by_tag/tag_"
# unique ID (here by tag)
id <- unique(data$tag)
# loop to make plots for all
foreach(i = id) %do% {
# subset data
data_subset <- data[tag == i]
# plot data
p <- atl_check_tag(
data_subset,
option = "datetime",
highlight_first = TRUE, highlight_last = TRUE
)
# save
agg_png(
filename = paste0(path, i, ".png"),
width = 3840, height = 2160, units = "px", res = 300
)
print(p)
dev.off()
}
Plot and save data in parallel loop
We can use the same structure, simply replacing %do%
with %dofuture%
, which is the key advantage of using
foreach.
The only additional step is setting up parallel
processing with registerDoFuture()
and
plan(multisession)
.
# load example data
data <- data_example
# file path
path <- "./outputs/maps_by_tag/tag_"
# unique ID (here by tag)
id <- unique(data$tag)
# register cores and backend for parallel processing
registerDoFuture()
plan(multisession)
# loop to make plots for all
foreach(i = id) %dofuture% {
# subset data
data_subset <- data[tag == i]
# plot data
p <- atl_check_tag(
data_subset,
option = "datetime",
highlight_first = TRUE, highlight_last = TRUE
)
# save
agg_png(
filename = paste0(path, i, ".png"),
width = 3840, height = 2160, units = "px", res = 300
)
print(p)
dev.off()
}
# close parallel workers
plan(sequential)